Components and Props:
Components are one of the most powerful features of Vue. They help you extend basic HTML elements to encapsulate reusable code.
1. Create a new component:
src/components/UserCard.vue:
vue create day2-sample
When prompted, select Vue 3.
Navigate into your project:
cd day2-sample
2. Install Vue Router:
npm install vue-router@next
3. Set up Vue Router:
src/router/index.js:
import { createRouter, createWebHistory } from 'vue-router'
import Home from '../views/Home.vue'
import Profile from '../views/Profile.vue'
const routes = [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/profile/:username',
name: 'Profile',
component: Profile,
props: true
}
]
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
4. Create views:
src/views/Home.vue:
<template>
<div>
<h2>Home Page</h2>
<p>Welcome to the Vue 3 app!</p>
<router-link :to="{ name: 'Profile', params: { username: 'john_doe' } }">Go to John Doe's Profile</router-link>
</div>
</template>
<script>
export default {
name: 'Home'
}
</script>
src/views/Profile.vue:
<template>
<div>
<h2>{{ username }}'s Profile</h2>
<p>This is {{ username }}'s profile page.</p>
</div>
</template>
<script>
export default {
props: {
username: String
}
}
</script>
5. Modify App.vue:
src/App.vue:
<template>
<div id="app">
<h1>Day 2 Vue 3 Sample App</h1>
<router-view />
</div>
</template>
<script>
export default {
name: 'App'
}
</script>
<style>
/* Some basic styling for visualization */
h1 {
background-color: #4CAF50;
color: white;
text-align: center;
padding: 1rem 0;
}
</style>
6. Modify main.js:
src/main.js:
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
createApp(App).use(router).mount('#app')
7. Run the App:
npm run serve
Now, you should have a Vue 3 app that has:
- A home page.
- A dynamic profile page, based on the username provided in the route.
Navigate to the home page, and then click on the link to navigate to the profile page. This demonstrates the Vue Router’s capability to handle dynamic routes.
This sample code introduces the basic concept of Vue Router in Vue 3, with a dynamic segment in the route (the username) which is passed as a prop to the profile component.
View in github branch https://github.com/LeoReyesDev/vue-tutorial/tree/day2-sample
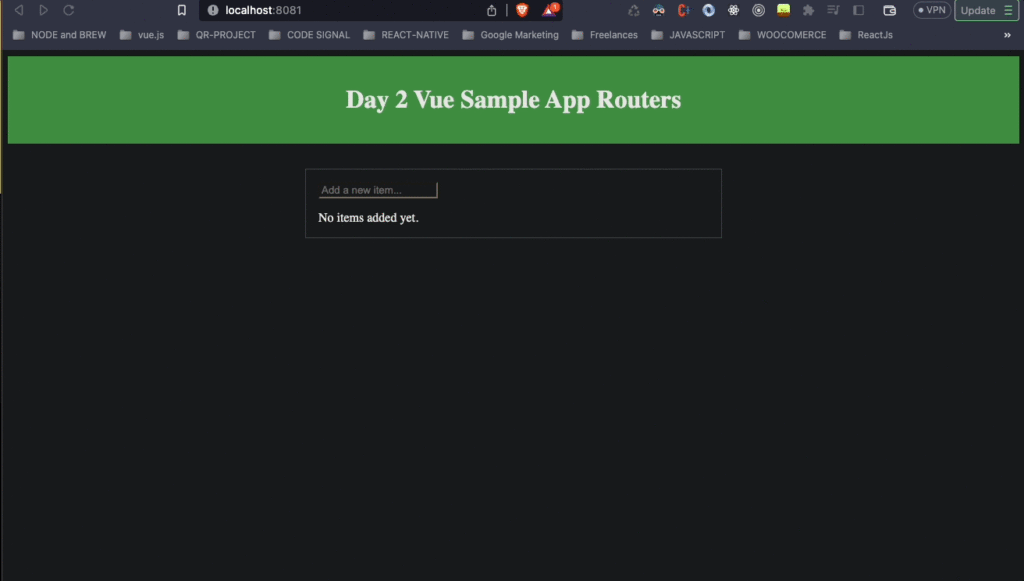